OpenCV와 PIL의 이미지 형태
- 결과를 보시면 알겠지만 width, height이 반대입니다.
import cv2
from PIL import Image
img = cv2.imread('Resources/cards.jpg')
h, w, c = img.shape
print(img.shape)
pil_img = Image.open('Resources/cards.jpg')
w, h = pil_img
print(a.size)
openCV 사진 좌표 형태
- openCV에서 좌표는 아래 사진처럼 표현 됩니다.
x축
은 오른쪽으로 가지만 y축
은 아래로 가는게 중요합니다.
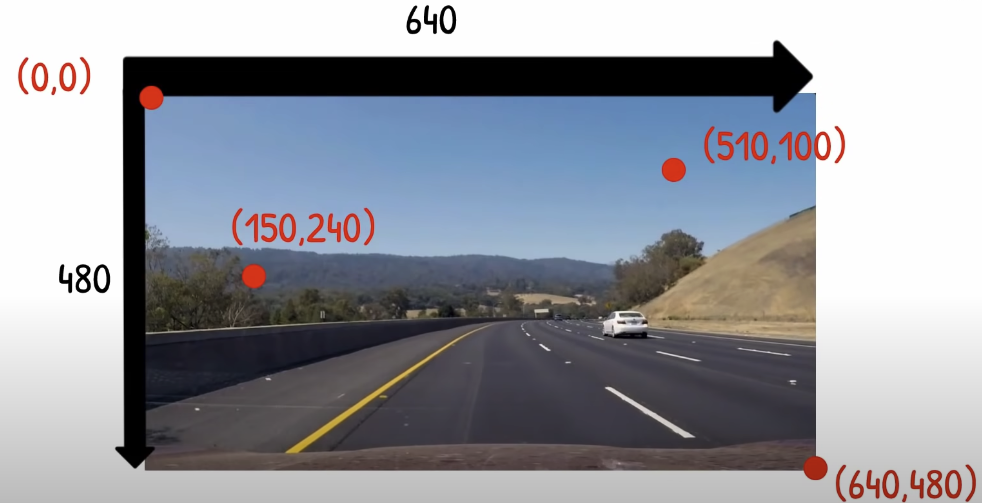
Resize
import cv2
img = cv2.imread('lena.png')
print(img.shape)
imgResize = cv2.resize(img,(200,150))
print(imgResize.shape)
cv2.imshow('origin',img)
cv2.imshow('resize',imgResize)
cv2.waitKey(0)
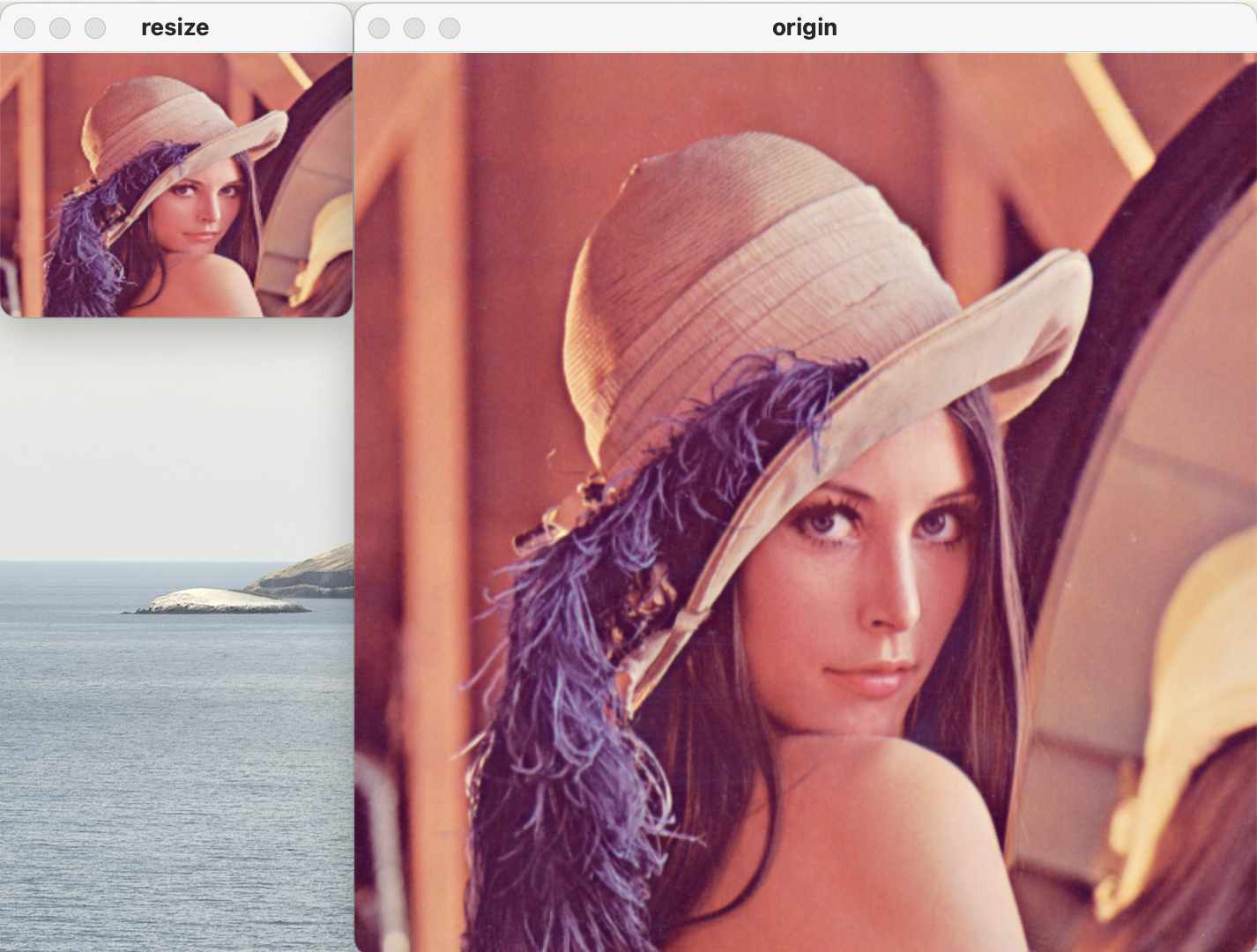
Crop
import cv2
img = cv2.imread('lena.png')
print(img.shape)
h, w, c = img.shape
imgResize = cv2.resize(img,(500,400))
print(imgResize.shape)
imgCropped = img[0:200,200:400]
cv2.imshow('resize',imgResize)
cv2.imshow('cropped',imgCropped)
cv2.waitKey(0)
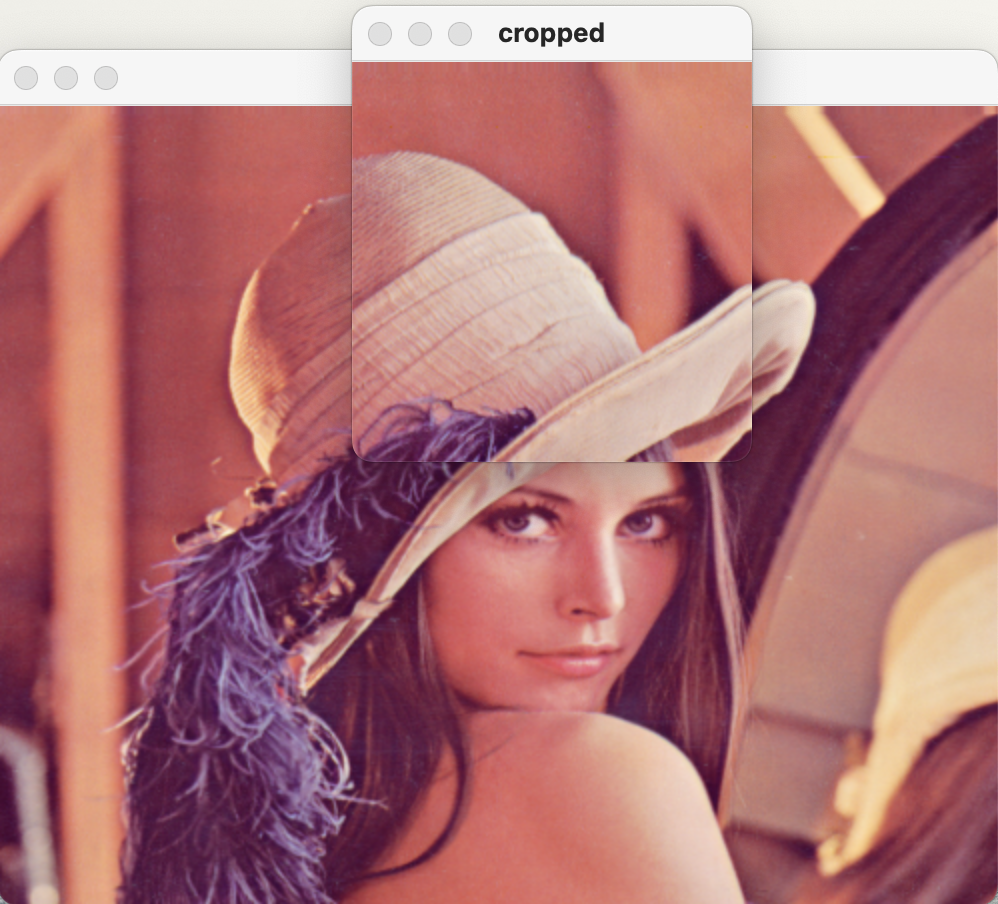
Drawing
import cv2
img = np.zeros((512,512,3), np.uint8)
img[200:300, 100:500] = 255,0,0
cv2.imshow('image1', img)
cv2.waitKey(0)
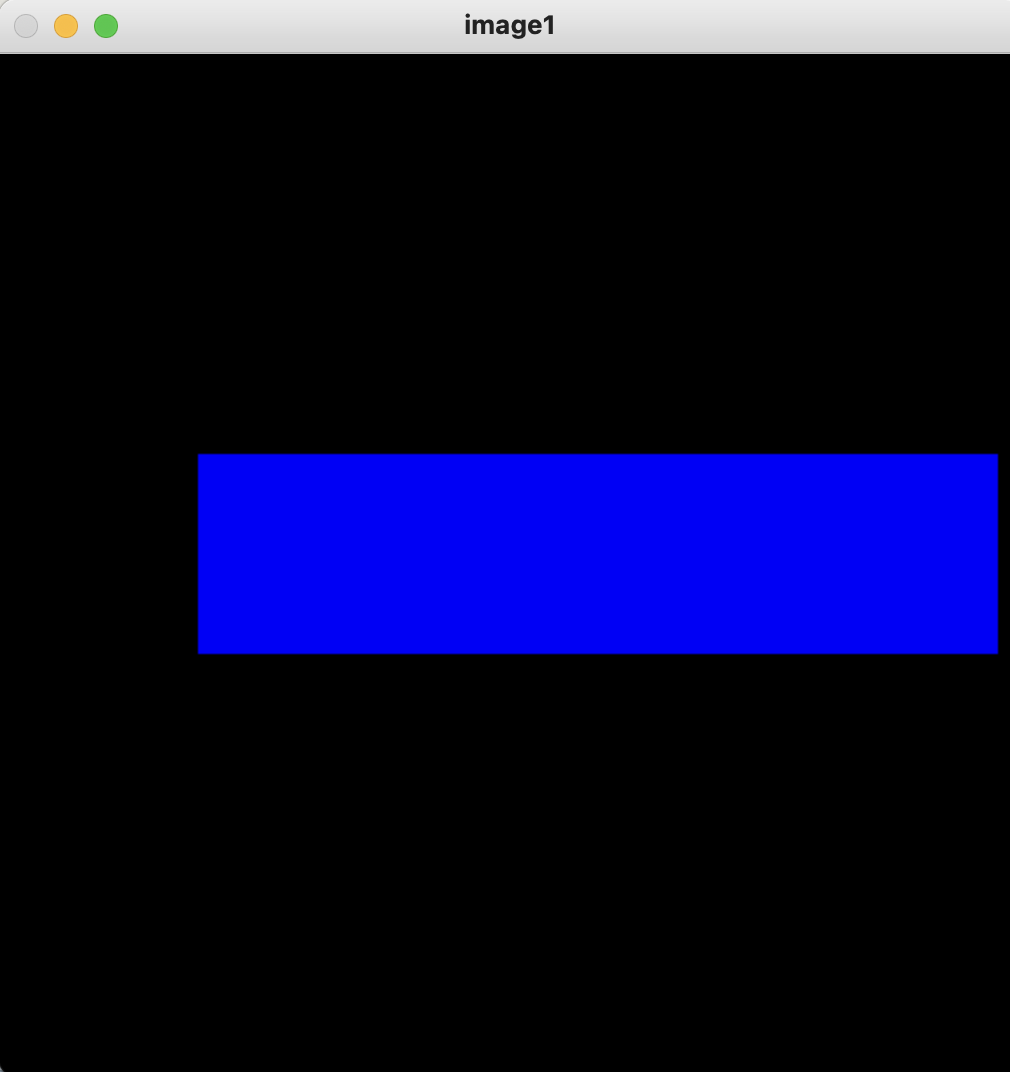
img = np.zeros((512,512,3), np.uint8)
cv2.line(img,(0,0),(300,300),color=(0,0,255),thickness=3)
cv2.imshow('image1', img)
cv2.waitKey(0)
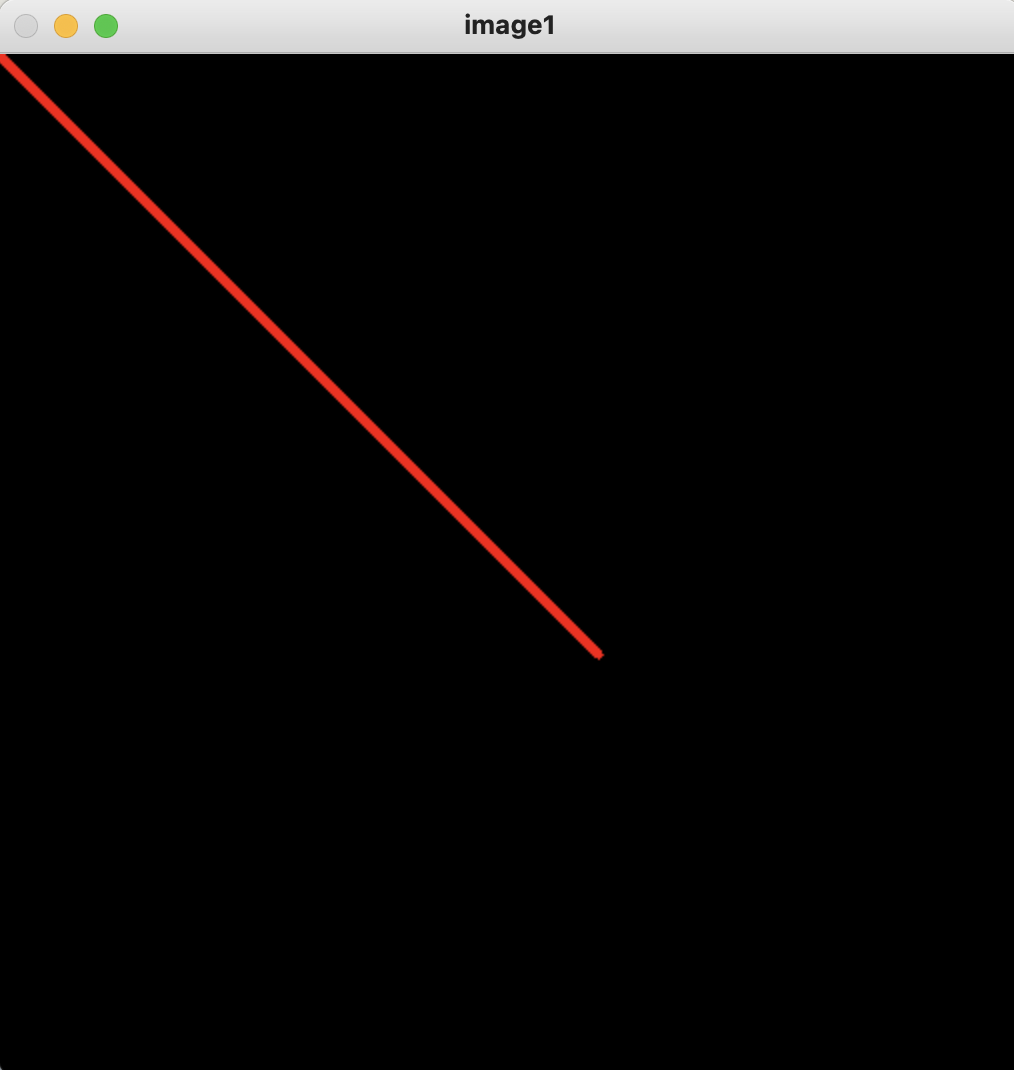
cv2.rectangle(img,(50,50),(500,200),(0,255,0),3)
cv2.imshow('image1', img)
cv2.waitKey(0)
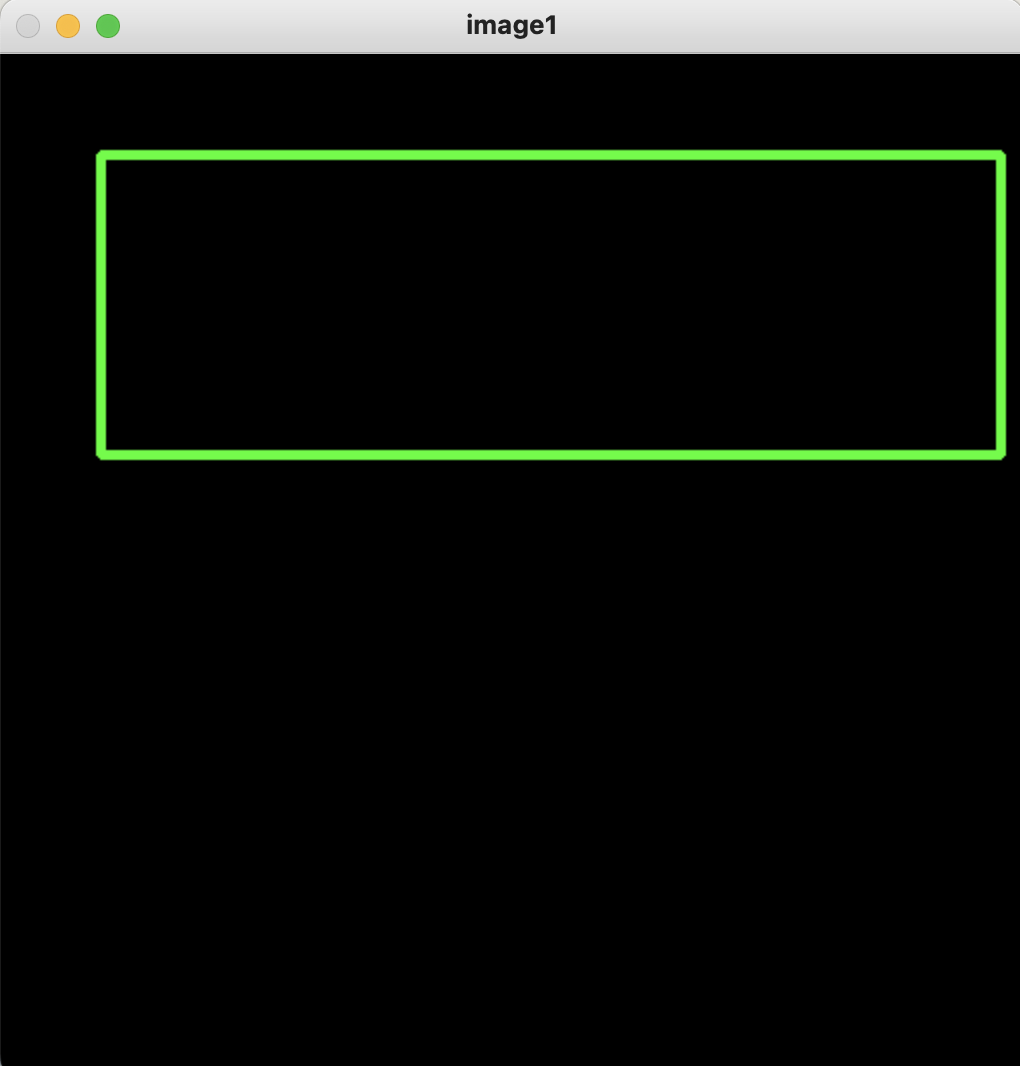
cv2.circle(img,(200,200), 30, (255,20,50),thickness=3)
cv2.imshow('image1', img)
cv2.waitKey(0)
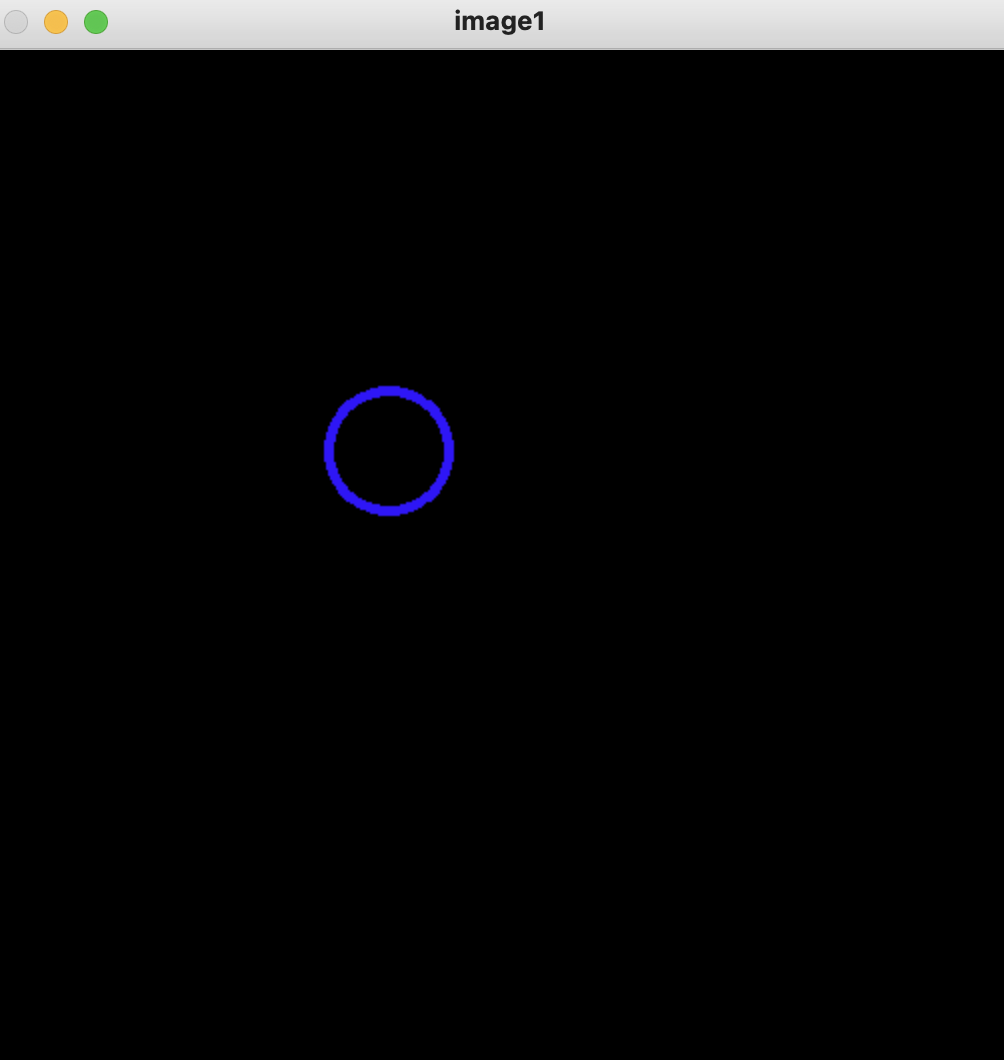
cv2.putText(img, 'THIS IS Text', (50,300),fontFace=cv2.FONT_HERSHEY_COMPLEX,fontScale=1,
color=(255,0,0),thickness=5)
cv2.imshow('image1', img)
cv2.waitKey(0)
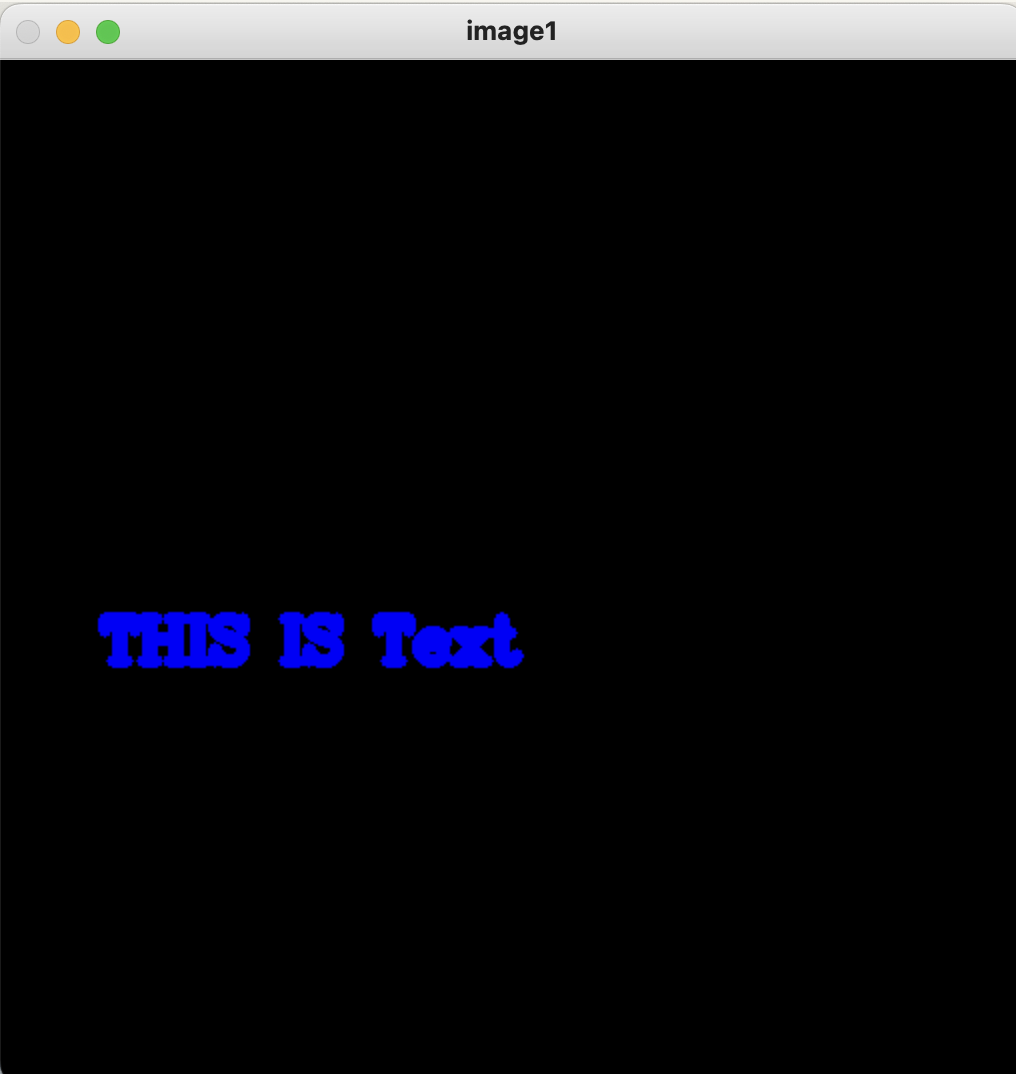